Plotting physt histograms¶
Some matplotlib-based plotting examples, with no exhaustive documentation.
[1]:
# Necessary import evil
import physt
from physt import h1, h2, histogramdd
from physt.plotting import matplotlib
import numpy as np
import matplotlib.pyplot as plt
# %matplotlib inline
np.random.seed(42)
from physt import plotting
[2]:
# Some data
x = np.random.normal(100, 1, 10000)
y = np.random.normal(10, 10, 10000)
[3]:
ax = h2(x, y, 15).plot(figsize=(6, 6), show_zero=False, alpha=0, text_color="black", show_values=True, cmap="BuGn_r", show_colorbar=False, transform=lambda x:1)
h2(x, y, 50).plot.image(cmap="Spectral_r", alpha=0.75, ax=ax)
[3]:
<AxesSubplot:xlabel='axis0', ylabel='axis1'>
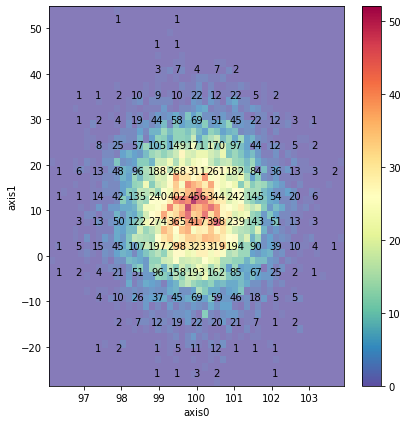
[ ]:
[4]:
h2(x, y, 40, name="Gauss").plot("image", cmap="rainbow", figsize=(5, 5))
[4]:
<AxesSubplot:title={'center':'Gauss'}, xlabel='axis0', ylabel='axis1'>
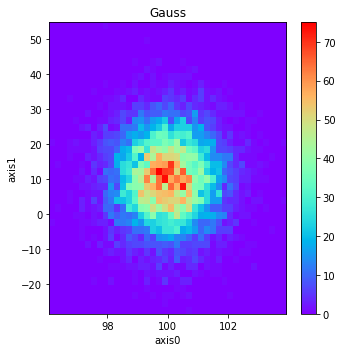
[5]:
plotting.matplotlib.bar3d(h2(x, y, 10, name="Gauss"), figsize=(5, 5), cmap="Accent");
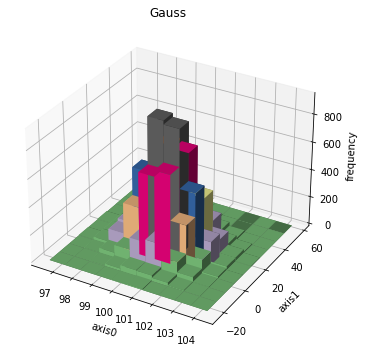
[6]:
h1(x, "human", bin_count=10, name="Gauss").plot(ylim=(100, 1020), cmap="Greys", ticks="edge", errors=True);
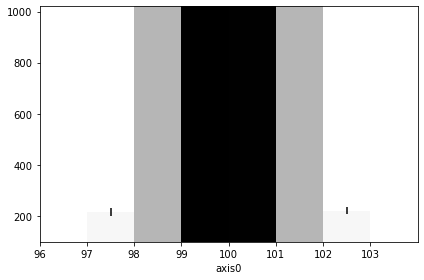
[7]:
h1(x, "human", bin_count=200, name="Gauss").plot.line(errors=True, yscale="log")
[7]:
<AxesSubplot:xlabel='axis0'>
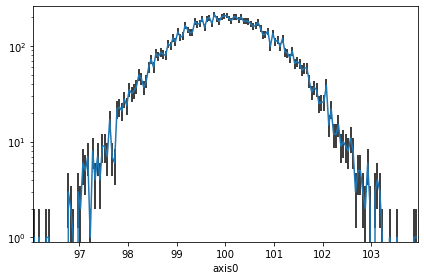
[8]:
h1(x, "human", bin_count=200, name="Gauss").plot.fill(lw=1, alpha=.4, figsize=(8, 4))
h1(x, "human", bin_count=200, name="Gauss").plot.fill(lw=1, alpha=.4, yscale="log", figsize=(8, 4), color="red")
[8]:
<AxesSubplot:xlabel='axis0'>
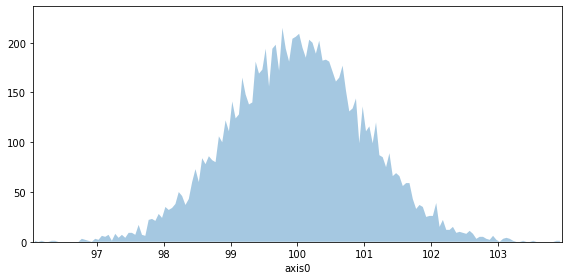
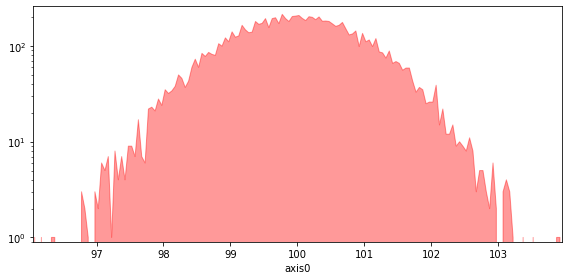
[9]:
h1(x, "human", bin_count=200, name="Gauss").plot.scatter(errors=True, xlim=(90, 100), show_stats="all")
[9]:
<AxesSubplot:xlabel='axis0'>
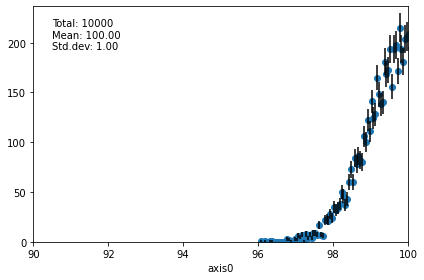
[10]:
ha = h1(x, "human", bin_count=20, name="Left")
hb = h1(x + 1 * np.sin(x / 12), "human", bin_count=40, name="Right")
from physt.plotting.matplotlib import pair_bars
[11]:
pair_bars(ha, hb, density=True, errors=True, figsize=(5, 5));
c:\users\janpi\documents\code\my\physt\physt\histogram_base.py:355: UserWarning:
Negative frequencies in the histogram.
c:\users\janpi\documents\code\my\physt\physt\plotting\matplotlib.py:789: UserWarning:
FixedFormatter should only be used together with FixedLocator
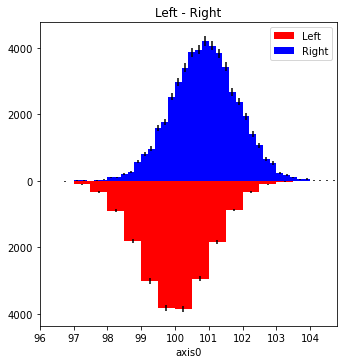
Example - histogram of time values¶
[12]:
# Get values close to
from physt.plotting.common import TimeTickHandler
data = np.random.normal(3600, 900, 4800)
H = h1(data, "human", axis_name="time")
H.plot(tick_handler=TimeTickHandler())
[12]:
<AxesSubplot:xlabel='time'>
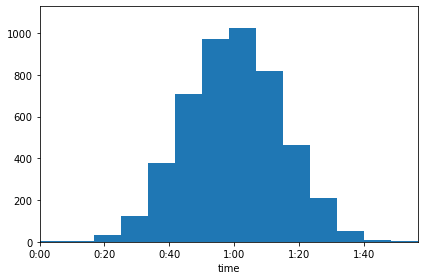